So you’ve just called your first REST service and have JSON array full of data… Where do you put it? I noticed lots of people using various plug-ins to create HTML tables by defining columns, data types, etc… but what happens when you don’t know this? Since I wanted to throw random JSON data into HTML tables, I created two helper functions that will iterate over the data to create the views.
This code below is an improvement to my ad-hoc JavaScript solution I created a few weeks ago for a ASP.NET project, link below. As you will see below, it’s pretty easy to render a HTML table from a object array using plain JavaScript. Since most results are in a Table or Detail layout, I created 2 functions to return the data in either format. I also added some optional parameters that you can set to control formatting. The Details View was designed to show a single row/object, the headers will display on the left and the data will display on the right (see second example).
// This function creates a standard table with column/rows
// Parameter Information
// objArray = Anytype of object array, like JSON results
// theme (optional) = A css class to add to the table (e.g. <table class="<theme>">
// enableHeader (optional) = Controls if you want to hide/show, default is show
function CreateTableView(objArray, theme, enableHeader) {
// set optional theme parameter
if (theme === undefined) {
theme = 'mediumTable'; //default theme
}
if (enableHeader === undefined) {
enableHeader = true; //default enable headers
}
// If the returned data is an object do nothing, else try to parse
var array = typeof objArray != 'object' ? JSON.parse(objArray) : objArray;
var str = '<table class="' + theme + '">';
// table head
if (enableHeader) {
str += '<thead><tr>';
for (var index in array[0]) {
str += '<th scope="col">' + index + '</th>';
}
str += '</tr></thead>';
}
// table body
str += '<tbody>';
for (var i = 0; i < array.length; i++) {
str += (i % 2 == 0) ? '<tr class="alt">' : '<tr>';
for (var index in array[i]) {
str += '<td>' + array[i][index] + '</td>';
}
str += '</tr>';
}
str += '</tbody>'
str += '</table>';
return str;
}
// This function creates a details view table with column 1 as the header and column 2 as the details
// Parameter Information
// objArray = Anytype of object array, like JSON results
// theme (optional) = A css class to add to the table (e.g. <table class="<theme>">
// enableHeader (optional) = Controls if you want to hide/show, default is show
function CreateDetailView(objArray, theme, enableHeader) {
// set optional theme parameter
if (theme === undefined) {
theme = 'mediumTable'; //default theme
}
if (enableHeader === undefined) {
enableHeader = true; //default enable headers
}
// If the returned data is an object do nothing, else try to parse
var array = typeof objArray != 'object' ? JSON.parse(objArray) : objArray;
var str = '<table class="' + theme + '">';
str += '<tbody>';
for (var i = 0; i < array.length; i++) {
var row = 0;
for (var index in array[i]) {
str += (row % 2 == 0) ? '<tr class="alt">' : '<tr>';
if (enableHeader) {
str += '<th scope="row">' + index + '</th>';
}
str += '<td>' + array[i][index] + '</td>';
str += '</tr>';
row++;
}
}
str += '</tbody>'
str += '</table>';
return str;
}
Standard Table Example Usage:
$(document).ready(function() {
$.ajax({
type: "POST",
url: "/SampleRestService",
contentType: "application/json; charset=utf-8",
dataType: "json",
data: "{}",
success: function(res) {
$('#Results').append(CreateTableView(res)).fadeIn();
}
});
});
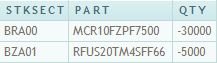
Details View Example Usage:
$(document).ready(function() {
$.ajax({
type: "POST",
url: "/SampleRestService",
contentType: "application/json; charset=utf-8",
dataType: "json",
data: "{}",
success: function(res) {
$('#Results').append(CreateDetailView(res,"CoolTableTheme",true)).fadeIn();
}
});
});

JSON to HTML JavaScript Source Code
That’s it, just do you normal jQuery ajax call and you can put your JSON into a HTML Table. This is a great way to test your JSON objects. I’ve tested the JSON to HTML on various object(s) and the results have been pretty good. If you find you need advanced features when your building your HTML table, you should look at something like jqGrid that has paging, sorting, etc…
[Update 10/6/2010]
A lot of people have asked for a full working HTML demo using JSON strings. The link below is a demo showing how to use the helpers with various JSON strings. Let me know if this helps!
#1 by Nick on July 28, 2014 - 8:17 am
thank you for the quick responce sorry if i dident make myself very clear. im trying to make it so that i can render the whole json data and view all the contents. curently it will just make one table give me the title field_ds_personal_details and a value of [object Object]. idealy in the value cell it would create another table containing that json data ending up with multyple tables.
field_ds_personal_details (table 1 cell 1)
instance_0 (new table(2) created in table 1 cell 2)
field_personal_faname (new table(3) created in table 2 cell 2)
value:Doe (new table(4) created in table 3 cell 2)
field_personal_fname (table 3 row 2)
vale: John( new table (5) created in table 3 row 2 cell 2)
( i may have my rows and cells mixed up a bit there but that should only effect the orientation and still explain it a bit better)
i experimented with editing the json.htm table.js file specficly
str += ” + array[i][index] + ”;
to make it check for an array or object and if there was one to run the generate table function again with that data to make a table in the cell. this did work for a randomly generated json file i got online but for my data it just gives me 1 table title field_ds_personal_details and the value cell being empty.
#2 by Zach on July 28, 2014 - 8:37 am
Your solutions sounds right, that’s exactly what I would do… I suggest putting a break point on the line of code where it tries to render the inner table (inside your IF statement), to see what the variable looks like. This is pretty easy with Firebug for FF, just open the window, enable script debugging, and put a breakpoint on the line. When the line is executed, you’ll be able to inspect the variables and see what’s breaking.
#3 by Nick on July 28, 2014 - 1:56 am
Hi Zach i realise this is a 4 year old post but you replied to a coment earler this year so i thought id try as well. i have some nested data in my json and it appears in the table as [object Object], [object Object] i was hopeing you could give me some help sorting this out as im haveing some trouble ( im prity new to all this not much prior knoladge). my json data is this:
[{“field_ds_personal_details”:{“instance_0”:{“field_personal_faname”:{“value”:”Doe”},”field_personal_fname”:{“value”:”John”},”field_personal_gender”:{“value”:”Male”},”field_personal_maname”:{“value”:””},”field_personal_mname”:{“value”:””},”field_personal_nickname”:{“value”:””},”field_personal_suffix”:{“value”:””},”field_personal_title”:{“value”:”Mr”},”field_personal_mobile_phone”:{“value”:”07430 296249″},”field_personal_work_phone”:{“value”:””},”field_personal_marital_status”:{“value”:”- Select -“},”field_personal_number_children”:{“value”:””},”field_personal_home_phone”:{“value”:”01999017535″},”field_personal_email_address”:{“value”:””}}}}]
thank you very much
#4 by Zach on July 28, 2014 - 7:37 am
What do you want/expect to happen with the object posted in your example. If you want to render the inner object (“field_ds_personal_details”), just pass it to the table function. I’m not sure what your expecting as your end results, so I’m not sure where to point you. So for example, if your $.ajax() method returned “results”, you could call
$('#resultsTable').append(CreateeTableViews(results.fields_ds_personal_details));
#5 by rfribeiro85 on May 9, 2014 - 3:54 am
Hi Zach,
I’ve been struggling with this for many many hours!
I’m not a developer, but, i have this need to get json data from my systems into a fancy table (and here’s your great tutorial)
I can make it work if i affect the json1 variable with this :
var json1 = { “d”: [{ host: “C010B0059001”, service_description: “Internet Explorer”, svc_plugin_output: “CRITICAL – Socket timeout after 10 seconds”, svc_state_age: “2014-02-19 17:53:12”, svc_check_age: “27 min”, service_icons: “”, perfometer: “”, svc_custom_notes: “”}] }
But, i want it to feed from a json file as this :
$(document).ready(function() {
$.ajax({
type: “POST”,
url: “new.json”,
contentType: “application/json; charset=utf-8”,
dataType: “json”,
data: “{}”,
success: function(res) {
$(‘#Results’).append(CreateDetailView(res)).fadeIn();
}
});
$(‘#DynamicGridLoading’).hide();
})
And my json file looks like :
{ “d”: [{ host: “C010B0059001”, service_description: “Internet Explorer”, svc_plugin_output: “CRITICAL – Socket timeout after 10 seconds”, svc_state_age: “2014-02-19 17:53:12”, svc_check_age: “27 min”, service_icons: “”, perfometer: “”, svc_custom_notes: “”}] }
And nothing comes up :X
Please remind that i’m not a developer, so sorry if i’m making stupid questions! :$
#6 by Zach on May 9, 2014 - 10:07 am
Your object data is wrapped in property “d”, you need to change “res” to “res.d” and it should work.
#7 by Tal on January 10, 2013 - 4:57 pm
I’m using your complete html project, but am instead replacing the var json1 with a live ajax proxy call to an external URL feed.
#8 by Tal on January 10, 2013 - 11:37 am
So currently this information below is displaying correctly, but now I’m trying to replace this dummy data from here
/* ASSOC ARRAY – Detail View */
var json1 = { “d”:[{“my json data}*/
with the url returned from the ajax proxy call
#9 by Tal on January 10, 2013 - 11:22 am
Thanks. I’m going the proxy route. So I’ve got the proxy.php in the root and am getting a successful call back with my json feed from my domain. Now I’m stuck applying the call to the var json1 variable here…
$.ajax({
url: ‘proxy.php?route=index.php/restofmyurl?limit=10’,
dataType: ‘json’,
success: function(dataObj, textStatus, jqXHR) {
createLeaderboard(dataObj);
},
});
#10 by Tal Thompson on January 5, 2013 - 7:31 pm
This might be obvious but how would I use a live URL json feed to be declared as the json1 variable?
#11 by Zach on January 7, 2013 - 9:42 am
I’m not following your question, the example shows pulling live data from a JSON feed via a AJAX call. This is the most common way to pull in live data from a JSON feed, if your pulling cross domain (different website), you’ll need to look at using JSONP or writing a proxy on the server to retrieve the data.
#12 by Dani on May 10, 2012 - 5:06 am
Hi Zach-
Thanks for this code. It really helped me a lot with a project I’m working on, and I’m quite grateful.
A question: I’m pulling data from several different JSONs and filtering them into your table. I’d like to create an error message for instances where there is no data available. I’ve added this after your for loop:
for (var i = 1; i > array.length;) {
str += (i % 2 == 0) ? ” : ”;
str+= ‘Data can not be found for your selected filters.’;
}
str += ”
str += ”;
return str;
The result is that all selections with data work perfectly, but selections without data just freezes the page. How would you deal with this issue?
Thanks so much, Zach!!
#13 by Zach on May 10, 2012 - 7:37 am
Without seeing the full code I’m not sure why it would hang, in general a jQuery ajax() call should never hang unless you set “async: false”. As for setting an error message based on your filtered data results, you could either show a message before you render the table (e.g. if filtered object is null, then show div). If you want to show a row saying no data, you’ll also have to determine the colspan of the TD so the message stretches across the entire table. If you can simulate your error with jsFiddle, post a link and I’ll take a look at it. Good Luck!
#14 by David on April 22, 2012 - 2:00 am
Not that it’s relevant anymore, but Gregory’s FastFrag is a small and incredibly awesome library to do just that. And it’s awesome.
#15 by Alex on April 15, 2012 - 8:56 am
Hey Zach, I just wanted to drop by and say thank you for this.
I have a little system that renders the logs of the phonecalls that went through our SIP server. I visualized it as a plain-text table, but it still looked pretty ugly.
I took your JavaScript thingie and adapted my Python script to generate the output in the format wanted by JS.
Now I have a pretty, zebrified table with active row highlighting. Yaay! 🙂
#16 by Zach on April 16, 2012 - 9:34 am
Alex, no problem… Glad it was helpful, I use the script all the time since it’s such a nifty block of code for making JSON legible..
#17 by Zach on March 23, 2012 - 4:44 pm
Possible yes, but not based on the code above. You’ll need to create something custom to parse your JSON and put it into the desired format (maybe a UL/LI structure). This is really easy to do, here is an example:
JavaScript Code
Basic HTML Layout using a UL.
Text Output of Code Above
#18 by Harpreet on March 8, 2012 - 9:45 am
excellent tutorial,
The code worked fine…. but i have a small issue… my json data is combination of say team_name and team_member_name, so each team has multiple members, so the code works fine which shows team_name : team_member_name, combination but i want that team_name to be displayed once and then team_member_names below that .. is it possible… i tried multiple things nothing works… am a new bee and its looking fro any help……
——————————–|
team_name1 | team_member_name1 |
——————————–|
team_name1 | team_member_name2 |
——————————–|
team_name1 | team_member_name2 |
——————————–|
team_name2 | team_member_name1 |
——————————–|
team_name2 | team_member_name2 |
——————————–|
team_name2 | team_member_name3 |
——————————–|
The way i want is:—
———————————-
team_name1|team_name2|team_name3|
———————————-
team_member_name1|team_member_name1|team_member_name1|
team_member_name2|team_member_name2|team_member_name2|
team_member_name3|team_member_name3|team_member_name3|
Please help
#19 by Ivan on January 6, 2012 - 11:12 am
Hi, great job !! …. i´m looking for a plugins like jquery dForm … that use json to create html but thisone create only form and what i´m trying to do are web pages for my site using json … if someone have any idea i really aprreciate
#20 by Zach on January 11, 2012 - 4:30 pm
If you just want to build a web page (say fill in a page template), you could use the MS contributed jQuery.template() plug-in. This allows you to create a template and to fill it on the fly using data you load via AJAX/JSON. This gives you a nice way to separate your data/layout and it’s really easy to use. Lots of tutorials online.
#21 by Sabina on December 21, 2011 - 12:44 pm
Thanks Zach!!
#22 by Andaman on June 29, 2011 - 9:18 am
I don’t know know why html tags are not showing.
div id=#myform in the body tags
#23 by Zach on June 29, 2011 - 11:49 am
Andaman,
Sorry, I’m getting confused reading the comments inline… and assumed they were talking about the JSON to HTML. As for getting the form’s working, I tested dForm again last week and had no issues getting my JSON to FORM working. I tested a few elements and different attributes (generate DIV vs. FORM) and everything worked. I’m not sure where I dropped the code, let me see if I kept it… If so, I’ll post a new entry that shows dForms working using your JSON (or a slightly modified variant).
Zach
#24 by Zach on June 29, 2011 - 12:21 pm
Andaman, check this out… Hope this helps.
http://new.zachhunter.com/2011/06/json-to-html-form-using-jquery-dform-plug-in/
#25 by Andaman on June 29, 2011 - 9:17 am
Why isn’t the HTML tagging showing?
I have ” in the body tags to display the form in the browser.
#26 by Andaman on June 29, 2011 - 9:14 am
Zach, at the bottom of what article is the download? Please give URL.
I have it working, but everything is on one line. There are no line breaks. Why is that?
Milindain/Zach,
Here is my code:
Untitled Document
var formdata = {
“action”: “index.html”,
“method”: “get”,
“elements”:
[
{
“type”: “p”,
“html”: “You must login”
},
{
“name”: “username”,
“id”: “txt-username”,
“caption”: “Username”,
“type”: “text”,
“placeholder”: “E.g. u…@example.com”
},
{
“name”: “password”,
“caption”: “Password”,
“type”: “password”
},
{
“type”: “submit”,
“value”: “Login”
}
]
};
$(document).ready(function(){
$(“#myform”).buildForm(formdata);
});
#27 by milindain on June 29, 2011 - 6:07 am
Similar to Andaman,
I am also struggling to find out way to get it working. Can anybody please provide step by step guide to get it working.
#28 by Zach on June 29, 2011 - 8:18 am
milindain,
At the bottom of the article, there is a download of a complete “working” project. You can use this as a “LIVE” demo to figure out what is going on and to figure out how to use/apply. If you can’t get the demo working, let me know.
Zach
#29 by Zach on June 29, 2011 - 12:21 pm
Milindain, check this out. Hope this helps.
http://new.zachhunter.com/2011/06/json-to-html-form-using-jquery-dform-plug-in/
#30 by Andaman on June 22, 2011 - 1:02 pm
Well, I’m back. I guess I spoke to soon. I copy & paste your code from jsfiddle. Here it is:
I don’t know what I am doing wrong. I can not get this page to work in a browser. The page is totally blank. Here is the code:
Untitled Document
$(document).ready(function){
var formdata = {
“action”: “index.html”,
“method”: “get”,
“elements”:
[
{
“type”: “p”,
“html”: “You must login”
},
{
“name”: “username”,
“id”: “txt-username”,
“caption”: “Username”,
“type”: “text”,
“placeholder”: “E.g. user@example.com”
},
{
“name”: “password”,
“caption”: “Password”,
“type”: “password”
},
{
“type”: “submit”,
“value”: “Login”
}
]
};
$(“#myform”).buildForm(formdata);
});
PLEASE tell me what I am doing wrong. I have saved the .js files in the root directory as my html page called “myform.html”.
Thanks
#31 by Andaman on June 22, 2011 - 12:24 pm
Thanks, Zach. Just what I was looking for….. where to put the code to get the json that creates the html elements
you made my DAY
#32 by Andaman on June 21, 2011 - 12:49 pm
Hi, I’ve been trying all day to get the json code to run in my browser using dforms plugin to build the form elements. I don’t know how to “wire it up” to the json data so that the elements will build out. I’m missing a step and the examples at http://neyeon.com/p/jquery.dform/doc/files2/examples-txt.html, don’t show you how to use the json data to get the html result — it only shows you the html and the json, not how to hook them up together
Untitled Document
here it is as a separate file:
#33 by Zach on June 21, 2011 - 3:27 pm
Here is a working example on jsFiddle.
#34 by Andaman on June 21, 2011 - 7:22 am
Zach, thanks, this is just what I needed. You rock, man
#35 by Andaman on June 20, 2011 - 8:51 pm
Great article. I’m also new JSON. I’m going through various tutorials (using PURE and jtemplate), but this is the best one I’ve seen.
I’m trying to create html form elements (or fields) using a JSON, i.e.: a “dropdownlist” and a “textarea” using the attributes below. I don’t have actual data, I’m just using the following schema. I’ve learned how to convert a simple json to html, and your article creating a table is advanced. Is there a way to create this JSON data “smart” enough to generate the HTML form elements I listed above? Is there a jQuery plugin/code that should be used?
{
itemID : null,
title : null,
comments : null,
typeID : 015,
subtypeID : 124,
images : null,
attributes :
[{
label : ‘Courses’,
dataType : ‘string’,
possibleValues : null,
selectedValue : null,
maxLength : 30,
required : 1
},
{
label : ‘FT Student’,
dataType : ‘boolean’,
possibleValues : [‘Yes’,’No’],
selectedValue : null,
maxLength : null,
required : 0
}]
}
thanks very much
#36 by Zach on June 20, 2011 - 10:42 pm
Totally possible, you should check out this jQuery Dynamic Form Plug-In. I’ve used this a few times and your layout looks really close to what you need to make this work.
#37 by Munish on June 13, 2011 - 5:00 am
i was trying to convert a char array into LPCWSTR bt got some errors.could someone plz tell me how to convert a char array into LPCWSTR…..
example…
i was trying to do
char arr[50] = “hellooo”;
LPCWSTR lValue = (LPCWSTR)arr
the above statement working perfectly fine but the next statement
IWBEMCLASSOBJECT->get(lValue , 0 , &value , 0);
is giving error … the error is about memory location.. at &value
plz help me out…
#38 by Zach on June 13, 2011 - 9:34 am
The question is a bit off topic, but what your doing sounds wrong. Sounds like your dealing with a UNICODE to ASCII issue. Here is a post on SO about this issue, with good reasons on why you should NOT be trying to convert your strings.
#39 by Munish on May 31, 2011 - 2:11 am
thanxs…
#40 by Munish on May 29, 2011 - 10:42 pm
i need to know how to bind json data with birt chart…. a simple example of birt.. it can hav x and y axis to bind values
#41 by Zach on May 30, 2011 - 7:11 pm
Every charting library has it’s now syntax for binding data.. and every JS library is going to support binding to JSON & Arrays of data. You should post a detailed questions stating the charting library name/version you want to use an a example of your JSON output to http://www.stackoverflow.com to get detailed help. The SO community includes people with tons of experience in all development languages/tools/etc… It’s the best way to get quick “technical” help to a development issue.
#42 by Munish on May 27, 2011 - 3:53 am
I am new to BIRT(business intelligence and reporting technique)… I want a simple example in which we can bind json data to birt chart….. i tried a lot but dint get anythng…
#43 by Zach on May 27, 2011 - 8:08 am
There are a ton of charting libraries for JS that will consume JSON from your service(s). Here is a link that previews a bunch of charting packages for JavaScript.
#44 by Chad Brown on March 29, 2011 - 3:00 pm
Great idea .. I’ve found this jQuery plug-in that allows you to transform JSON data into HTML .. it’s called json2html: http://plugins.jquery.com/plugin-tags/json2html
#45 by jay on February 11, 2011 - 2:36 am
hi zach..,
i am new to json.. can i make jquery tab dynamically using javascript? and i want to make the get the title from the .json file… is it possible to do that? i can’t even do tabs dynamically in html? so as connecting the tabs title to the .json file. please help… thank you..
Pingback: Tweets that mention Convert JSON to HTML using JavaScript « Zach Hunter's Busy Life -- Topsy.com
#46 by tom perkins on January 11, 2011 - 8:28 am
hello there zach,
thanks for the codes above they are very helpfull. but i’m trying to get the data from a json feed
for example this link
http://api.erepublik.com/v2/feeds/citizens/664491.json
how can i change the $(document).ready part according to the link.
thanks for the answer. regards. tom
#47 by narsimhamudiraj on October 4, 2010 - 4:35 am
Hi please send me sample code in html table format using Json String.Thanks
#48 by Zach on October 6, 2010 - 8:38 am
Narsimha,
I’ve just added a full working HTML demo showing how to parse various JSON strings into a Details View and Table View. Here is the link http://new.zachhunter.com/wp-content/uploads/JsonToHTML.zip, you can also fit it at the bottom of the article now.
Hope this helps,
Zach
#49 by narsimhamudiraj on October 4, 2010 - 3:50 am
Hi, please send the complete code (including html) of above example
#50 by Steve on September 1, 2010 - 2:17 am
Just thinking, rather than building up strings why not create an array representing the table then use the join method. Apparantly its more efficient than concatenation. I will be testing this against a rather large data set and will post the results for all who are interested.
#51 by Zach on September 1, 2010 - 11:47 am
It really depends on the applicaiton, but going from JSON to an array doesn’t make sense to me. I do agree that building a big table as a string could make the clients browser become unresponsive, I think a better solution is to write an empty table to then DOM and then add rows to the table as you loop over your source data.
Example:
$("some div").append("< table id=’dynamicTable’>< /table>");
— for each row in source data do
$(‘#dynamicTable tr:last’).after(‘< tr>< td>….< /td>< /tr>’);
** You could initially hide the table and show when load is complete.
#52 by Prabu on August 10, 2010 - 7:34 am
Please can you provide sample html code with example json String.So it will be more helpfull for me…
Thanks in Advance..
Regards
Prabu.N
#53 by Jeff on July 21, 2010 - 2:21 pm
Never mind I figured it out. Thanks for the great tutorial.
Just in case anyone is wondering… My JSON data was:
{“d”:[{“__type”:”myJQueryTestBed.names”,”name”:”Jeff”,”title”:null,”state”:”closed”},{“__type”:”myJQueryTestBed.names”,”name”:”Steve”,”title”:null,”state”:”closed”},{“__type”:”myJQueryTestBed.names”,”name”:”JT”,”title”:null,”state”:”closed”}]}
I was able to get my data while looping by doing this:
array[i].name
array[i].state
#54 by Jeff on July 21, 2010 - 2:07 pm
When I alert out the array all I get is [object,object][object,object][object,object]
How do I drill into the array to get the appropriate data?
#55 by surendran on July 15, 2010 - 12:51 pm
I am new to JSON. I am looking for this kind of a table formating. I am not able to get this code work. Not sure What are the other API files to be included in the header of the html page.
It would be great if you could publish a complete working example with some sample JSON data
thank you
#56 by Zach on July 20, 2010 - 2:28 pm
Sunrendran,
Sorry you were not able to get the code working, the only thing required to use these functions is jQuery and a JSON string or JavaScript object. If your not familiar with either, it’d probably be best to create a simple example and then expand it out as you understand each new concept.
Here is an example using a JavaScript object (easy to understand):
var objArray = new Array();
var obj1 = new Object;
obj1.Name = 'Zach';
obj1.Email = 'Zachary.Hunter@gmail.com';
objArray.push(obj1);
var obj2 = new Object;
obj2.Name = 'Hcaz';
obj2.Email = 'gmail@Zachary.Hunter.com';
objArray.push(obj2);
$('#results').append(CreateDetailView(objArray));
#57 by AneeshAbe on June 7, 2010 - 10:10 pm
Hi Zach,
It worked out well!
Thank you..
#58 by AneeshAbe on June 7, 2010 - 1:48 pm
Hi Zach,
When I run the code I get my first header of table as ‘__type’ and content as ‘acation’ which is the object type. How can I remove the first element of the object array?
I tried with shift() but it was not successful. What do you suggest on this?
#59 by Zach on June 7, 2010 - 2:41 pm
AnneeshAbe,
I going to assume your still using the data in your previous example from the other day. The reason this will not work is because your data is stored in an associative array which is not support in JavaScript. If you want to delete an item from an associative array, use the following syntax ( ex: delete res.d[0][“__type”]; ). In my example, an $.ajax() call would return a JSON response called “res”.
Zach
#60 by AneeshAbe on June 2, 2010 - 11:04 pm
Hi Zach,
Your tip worked. Thanks.
However , we cant go forward with this approach for production level application. So I am relying on jtemplate plug in for a start.
As you said, jqGrid looks amazing!
#61 by AneeshAbe on June 1, 2010 - 3:13 pm
Hi ,
Your code looks very interesting.
I was trying to test this with my web service but it is getting hanged at var array = JSON.parse(objArray);
I was trying to make a details view.
At success event I am adding as:
$(‘#myDiv’).append(CreateDetailView(result, “CoolTableTheme”, true).fadeIn());
Somehow it is not working.
The json object I am getting from server look like thsi:
{“d”:[{“__type”:”acation”,”id”:”001″,”date”:”2010-06-01 00:00:00″,”iod”:”1″,”mer”:”ABC “,”tity”:”6″,”ot”:”12,500″,”nt”:”75000″,”ou”:”A”,”rep”:”we”,”perc”:”34″,”ine”:”one”,”year”:”2009″,”ct”:”ABC “,”alet”:”90000″,”pro”:”1500″,”stats”:”ive”,”crnt”:”5000″,”ter”:”AA”}]}
Could you please add an example json object so that we can run this code?
Your help will be appreciated much!
#62 by Zach on June 1, 2010 - 4:24 pm
The problem is the encoding of your JSON data, the data you posted above is being returned as an object versus a JSON string. To get around this, you just need to make one small change to the code. Here it is.
Before:
var array = JSON.parse(objArray);
After:
var array = typeof objArray != ‘object’ ? JSON.parse(objArray) : objArray;
I’ll update my example now with this change.
#63 by Krishna on May 20, 2010 - 11:25 am
Thanks a lot! I was looking for something similar. I am creating a simple client to query my semantic database and display the result. So the columns of the query result were dynamic(based on the query) and almost all the Jquery plugins were not helpful in my case.
Thanks Again Zach!!!